Laravelでユーザー登録時に確認メールを送る方法
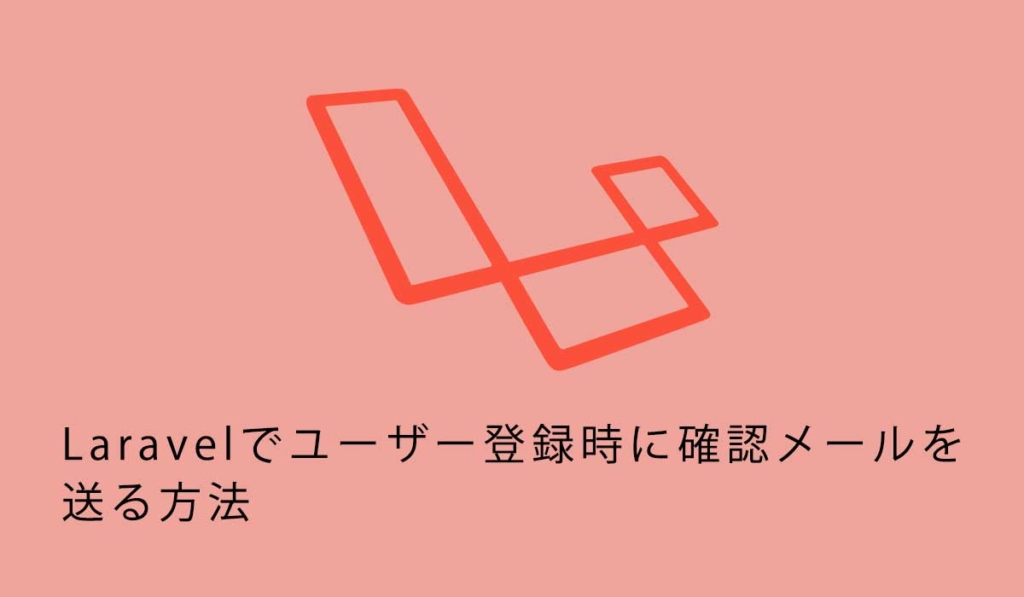
3033 回閲覧されました
みなさんこんにちは、jonioです。
今回はLaravelのBreeze等でログイン機能を作ってユーザー登録した時に確認のメールが来る様になる方法について解説します。
目次
Laravelのバージョン
バージョンは8です。
前提としてしないといけない事
ログイン機能をBreezeで実装するなら↓の記事を読んで実装して下さい。
またLaravelのプロジェクト直下にある「.env」ファイルにメールを送信する為の記述をしないといけないのですが↓の解説の「.envの編集」の項目を参考にしてやってみて下さい。
Userモデルの編集
「Laravelのプロジェクト > app >Models > User.php」を編集します。
まずはEmail認証を必須にする為の設定です。
↓の3つのuse文を追加します。
この3つの文はLaravelのバージョン8ではデフォルトで付いているみたいです。
次にUser.phpの14行目辺りにある「class User extends・・・」のコードを↓に編集します。
次はルーティングです。
ルーティング
web.phpを編集します。
「Auth::route(); 」がありますが「Auth::routes([‘verify’ => true]); 」に変えます。
更にメール認証をしてユーザー登録した場合だけアクセスする事ができるページの設定をweb.phpにします。(必須ではないです)
例えばユーザー登録をしたらトップページを見る事ができる様にする為のコードは↓みたいな感じです。
ここまでができたらアカウントを作るページに移動します。
↓のページで項目に入力して「アカウント作成」を押します。
するとメールアドレスの確認画面になります。
また、確認のメールが届きます。
メールアドレスの確認画面・確認メールは文章が少し硬い感じがするので内容を変えます。
メールアドレスの確認画面の修正
確認画面を表示するbladeは「Laravelのプロジェクト > resources > views/auth > verify.blade.php」ですがこれを修正します。
今の状態は↓です。
これを↓にします。
8行目・13行目・17行目・18行目・22行目を修正しています。
これで確認画面が↓に変わります。
次は確認メールの修正です。
確認メールの修正
確認メールの中身は「Laravelのプロジェクト > vendor > laravel > framework > src/Illuminate > Auth > Notifications > VerifyEmail.php」にあります。
65行目〜68行目の英語が日本語に翻訳されています。
日本語に翻訳するファイルは「Laravelのプロジェクト > resorces > lang > ja.json」 です。
例えばVerifyEmail.phpの65行目の「Verify Email Address」はja.jsonの738行目に翻訳があります。
日本語化をしてない方は↓の記事を読んでやってみて下さい。
ファイルの中身の変更はダメ
VerifyEmail.phpですが日本語に翻訳するのではなくファイルの中身を変更すればいいと考える方もいると思います。
でもそれはできません。
変更してはいけない理由
理由はvendorフォルダの中のファイルはライブラリ(プログラムを誰でも簡単に使える様にした物)で開発環境で変更しても本番環境に持って行く事ができないからです。
だからNotificationという機能を使ってVerifyEmail.phpを上書きして確認メールの内容を変えます。
Userモデルの編集
「Laravelのプロジェクト > app > Models > User.php」を編集します。
ファイルの中身の一番上にuse文がありますが↓を追加します。
また↓のメソッドを追加します。
新規Notificationの作成
ターミナルで↓のコマンドを叩きます。
すると「Laravelのプロジェクト > app > Notifications > NewVerifyEmail.php」が作成されます。
use文に↓を追加します。
また、43行目〜49行目辺りに↓のコードがあります。
これを↓に変えます。
これでユーザー登録をして確認メールを見ると↓に表示が変わります。
↑の「このボタンをクリック」を押すとデータベースのusersテーブルにメール認証をした日時も登録されます。
これで確認メールの修正が完了です。
「よろしくお願いします」の修正
確認メールの「よろしくお願いします,」がまだ修正されていません。
この部分の修正方法も解説します。
ターミナルで↓のコマンドを叩きます。
すると「Laravelのプロジェクト > resources > views > vendor > notifications > email.blade.php」が作成されます。
46行目辺りに「@lang(‘Regards’),」があるのでそれを変更すると 「よろしくお願いします,」を変える事ができます。
ロゴの修正
今の状態の確認メールはLaravelのロゴがあります。
これを変える事ができる様にします。
ターミナルで↓のコマンドを叩きます。
すると「Laravelのプロジェクト > resources > views > vendor > mail > html > header.blade.php」 が作成されます。
5行目辺りにimgタグがあります。
これを変更すればLaravelのロゴを変更する事ができます。