jQueryで画面をスクロールした時にヘッダーをフワッと表示する方法
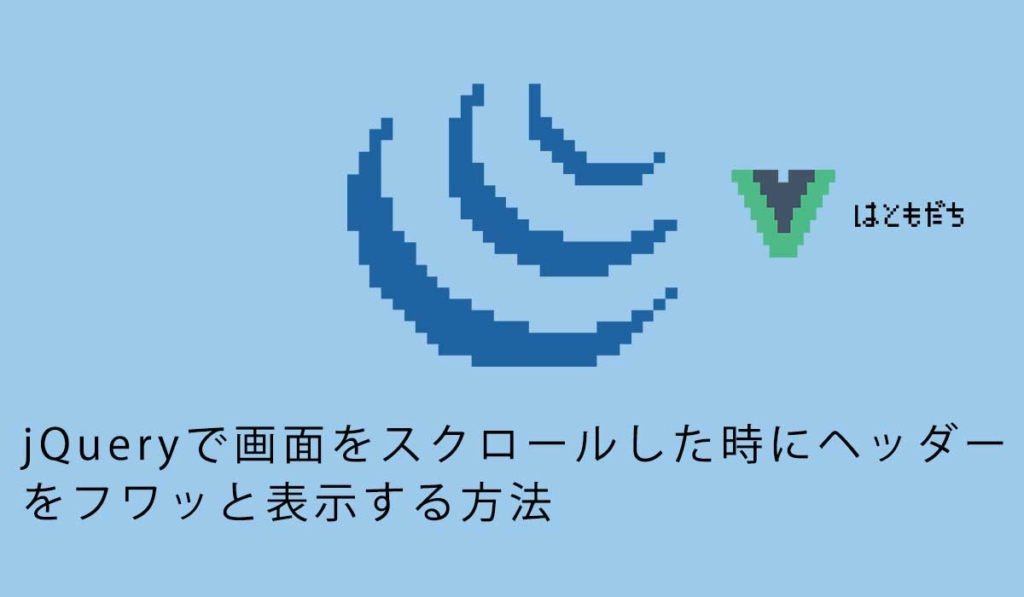
1896 回閲覧されました
みなさんこんにちは、jonioです。
今回は画面をスクロールした時にヘッダーをフワッと表示する方法を説明します。
デモ画面
デモは↓です。
See the Pen
Untitled by hayata19791218 (@hayata19791218)
on CodePen.
コードの解説
HTMLとcssを↓にします。
//HTML
<!DOCTYPE html>
<html lang="ja" dir="ltr">
<head>
<meta charset="utf-8">
<title>jQueryでフェードイン</title>
<link rel="stylesheet" href="css/style.css">
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
</head>
<body>
<header>
<nav>
<ul>
<li>ナビゲーション1</li>
<li>ナビゲーション2</li>
<li>ナビゲーション3</li>
<li>ナビゲーション4</li>
<li>ナビゲーション5</li>
</ul>
</nav>
</header>
<header id="header" class="header">
<nav>
<ul>
<li>ナビゲーション1</li>
<li>ナビゲーション2</li>
<li>ナビゲーション3</li>
<li>ナビゲーション4</li>
<li>ナビゲーション5</li>
</ul>
</nav>
</header>
<main>
<div class="content">コンテンツ</div>
</main>
<script type="text/javascript" src="js/common.js"></script>
</body>
</html>
//css
header ul{
display: flex;
justify-content:space-between;
width: 80%;
margin-left:auto;
margin-right:auto;
}
header nav{
background-color:skyblue;
padding-top: 20px;
padding-bottom: 20px;
}
header{
width: 100%;
}
.content{
height: 5000px;
background-color:pink;
}
.header{
position:fixed;
top:-70px;
transition-duration:1s;
}
.fadein{
top:0px;
}
/* ここからreset.css */
html, body, div, span, object, iframe,
h1, h2, h3, h4, h5, h6, p, blockquote, pre,
abbr, address, cite, code,
del, dfn, em, img, ins, kbd, q, samp,
small, strong, sub, sup, var,
b, i,
dl, dt, dd, ol, ul, li,
fieldset, form, label, legend,
table, caption, tbody, tfoot, thead, tr, th, td,
article, aside, canvas, details, figcaption, figure,
footer, header, hgroup, menu, nav, section, summary,
time, mark, audio, video {
margin:0;
padding:0;
border:0;
outline:0;
font-size:100%;
vertical-align:baseline;
background:transparent;
}
body {
line-height:1;
}
article,aside,details,figcaption,figure,
footer,header,hgroup,menu,nav,section {
display:block;
}
nav ul {
list-style:none;
}
blockquote, q {
quotes:none;
}
blockquote:before, blockquote:after,
q:before, q:after {
content:'';
content:none;
}
a {
margin:0;
padding:0;
font-size:100%;
vertical-align:baseline;
background:transparent;
text-decoration:none;
}
del {
text-decoration: line-through;
}
abbr[title], dfn[title] {
border-bottom:1px dotted;
cursor:help;
}
hr {
display:block;
height:1px;
border:0;
border-top:1px solid #cccccc;
margin:1em 0;
padding:0;
}
input, select {
vertical-align:middle;
}
p,.nav{
margin:0;
}
/* ここまでreset.css */
22行目〜32行目の部分がスクロールした時に上からフワッと表示されるヘッダーです。
61行目〜65行目でスクロールした時にフワッと表示するヘッダーが画面の外に隠れるようにしています。
jQuery
まずはスクロールした時に表示するヘッダーを取得します。
$(function(){
const header = $("#header");
});
次に画面をスクロールした時を考えます。
$(function(){
const header = $("#header");
//ここから追加
$(window).on("scroll",function(){
});
//ここまで追加
});
画面に対して何かをする時は6行目の「$(window)」です。
次はスクロール量を取得します。
$(function(){
const header = $("#header");
$(window).on("scroll",function(){
let scroll = $(window).scrollTop(); //この行を追加
});
});
4行目の「.scrollTop()」でスクロール量を取得でき「$(window).scrollTop()」で画面のスクロール量を取得できます。
次は画面をスクロールするとヘッダーが上からフワッと表示できるようにします。
$(function(){
const header = $("#header");
$(window).on("scroll",function(){
let scroll = $(window).scrollTop();
//ここから追加
if(scroll > 100){
header.addClass("fadein");
}else{
header.removeClass("fadein");
}
//ここまで追加
});
});
8行目〜10行目でスクロール量が100pxを越えたらfadeinクラス(top:0)が画面の上に隠れていたヘッダーに付いて画面上に表示されます。
10行目〜12行目でスクロール量が100pxより小さくなったらfadeinクラスが外れて画面上に戻ります。
これで完成です。