CakePHP3でPOST・GETの場合にjQueryでAjaxをしてうまくいく方法
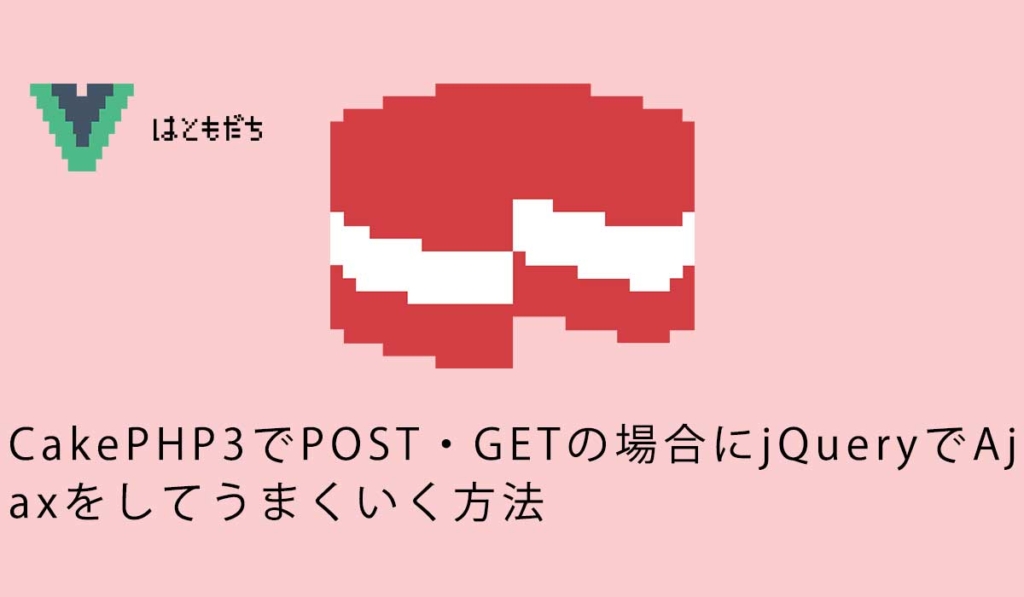
324 回閲覧されました
みなさんこんにちは、jonioです。
Ajaxを使っていてこういう流れで実装すれば色んな場合に対応できる方法が分からず悩んでいました。
ネットの記事を読むと人によって実装方法が違うので「どうすればいいんだろう?」とずっと思っていましたがPOSTとGETの場合にこう記述すればいいだろうという方法が分かったので解説します。
おすすめ参考書
CakePHP3はまだまだ仕事で使われます。
下記の参考書がおすすめです。
CakePHPのバージョン
3です。
GET
ボタンなどをクリックした時にusersテーブルからユーザー情報を取得して表示する場合を考えます。
jQueryの記述を下記にします。
$('#user-list').on('click', '.select-employee', function(e) {
e.preventDefault();
selectedId = $(this).data('id');
let userName = $(this).data('name');
let userNumber = $(this).data('number');
$.ajax({
url: '/url/demo-action?user_id='+ selectedId,
success: function(response) {
let list = '';
$.each(response, function(index, value) {
list += `<p>${value} ${userNumber} ${userName}</p>`
});
$('#wrap').html(list);
}
});
})
11行目の「?user_id=’+selectedId」で取得するユーザーのidをコントローラーに渡します。
この部分のコードの書き方は下記になります。
'/url/action名/?key=' + value
11行目が終わった時点でコントローラーに「?user_id=’+selectedId」のデータが渡ります。
一旦コントローラーに移ります。
コントローラーに下記の記述をします。
public function action()
{
$this->viewBuilder()->setLayout(false);
$this->render(false);
$response = $this->getResponse()->withCharset('UTF-8')->withType('json');
$this->setResponse($response);
$result = [];
$query = $this->Users->find();
if (!empty($this->getRequest()->getQuery('user_id'))) {
$query->where([
'Users.id' => $this->getRequest()->getQuery('user_id'),
'created LIKE' => date('Y-m-d').'%'
]);
}
$query->toArray();
foreach ($query as $data) {
$result[] = $data->name.' '.$data->stamp_date->format('H:i');
}
$response = $this->getResponse()
->withStringBody(json_encode($result, JSON_UNESCAPED_UNICODE));
$this->setResponse($response);
}
3行目4行目はアクションの動作時にテンプレートが通常必要ですがjson使用時にページを表示させる必要がないのでその為の設定です。
5行目で文字コードをUTF-8にして(いつも使っている文字を使えるようにすること)データの種類をjsonに設定します。
6行目があることで5行目の設定をAjaxのコードの12行目のresponse(下記参照)に返すことができます。
$.ajax({
url: '/url/demo-action?user_id='+ selectedId,
success: function(response) { //←のresponse
let list = '';
$.each(response, function(index, value) {
list += `<p>${value} ${userNumber} ${userName}</p>`
});
$('#wrap').html(list);
}
});
7行目〜18行目はコントローラーでusersテーブルから情報を取得する為の設定です。
9行目の「$this->getRequest()->getQuery(‘user_id’)」はAjaxの11行目(下記参照)のkeyです。
$.ajax({
url: '/url/demo-action?user_id='+ selectedId, //←のuser_idのこと
success: function(response) {
だから「$this->getRequest()->getQuery(‘user_id’)」の値は「selectedId」です。
19行目・20行目は17行目の$result(Ajaxに返すデータ)を文字コードがUTF-8でjsonデータとしてAjaxに返せるようになります。
そして21行目で$resultをAjaxに返します。
そして話をAjaxに戻します。
$('#user-list').on('click', '.select-employee', function(e) {
e.preventDefault();
selectedId = $(this).data('id');
let userName = $(this).data('name');
let userNumber = $(this).data('number');
$.ajax({
url: '/url/demo-action?user_id='+ selectedId,
success: function(response) { //←ここからの説明
let list = '';
$.each(response, function(index, value) {
list += `<p>${value} ${userNumber} ${userName}</p>`
});
$('#wrap').html(list);
}
});
})
13行目〜17行目はコントローラーから受け取った情報を表示する為のjQueryの記述です。
12行目の「response」の中にコントローラーから渡った情報が入っているのでこれを扱う為に12行目の下に「console.log(response)」を記述して情報がどんな構造になっているかを確認した方がいいです。
POST
ユーザー情報をusersテーブルに保存する場合を考えます。
jQueryの記述を下記にします。
$('#store').on('click', function() {
event.preventDefault();
$.ajax({
url: '/url/action?user_id=' + selectedId,
type: 'POST',
success: function(response) {
//ユーザー情報をテーブルに保存後の処理
}
})
})
GETの場合と違うのは6行目です。
これがないとエラーになります。
次はコントローラーですがAjaxを設定する為の記述は同じです。
public function action()
{
$this->viewBuilder()->setLayout(false);
$this->render(false);
$response = $this->getResponse()->withCharset('UTF-8')->withType('json');
$this->setResponse($response);
if ($this->getRequest()->is('post')) {
$user = $this->Users->newEntity();
$user->user_id = $this->getRequest()->getQuery('user_id');
$attendanceLog = $this->Users->patchEntity($user, $this->getRequest()->getData());
if ($this->Users->save($user)) {
$response = ['status' => 'success'];
}
$response = $this->getResponse()->withStringBody(json_encode($response, JSON_UNESCAPED_UNICODE));
$this->setResponse($response);
}
}
といった具合です。
ポイント
Ajaxは下記の2行目のコントローラーへの変数の渡し方だと思います。
$.ajax({
url: '/url/action?key='+ value,
success: function(response) {
//Ajax成功時の処理
}
});
コントローラーは下記です。
public function action()
{
$this->viewBuilder()->setLayout(false);
$this->render(false);
$response = $this->getResponse()->withCharset('UTF-8')->withType('json');
$this->setResponse($response);
$result = [];
//コントローラーでの処理
$response = $this->getResponse()
->withStringBody(json_encode($result, JSON_UNESCAPED_UNICODE));
$this->setResponse($response);
}