CakePHP3で複数認証(ログイン)を実装する方法
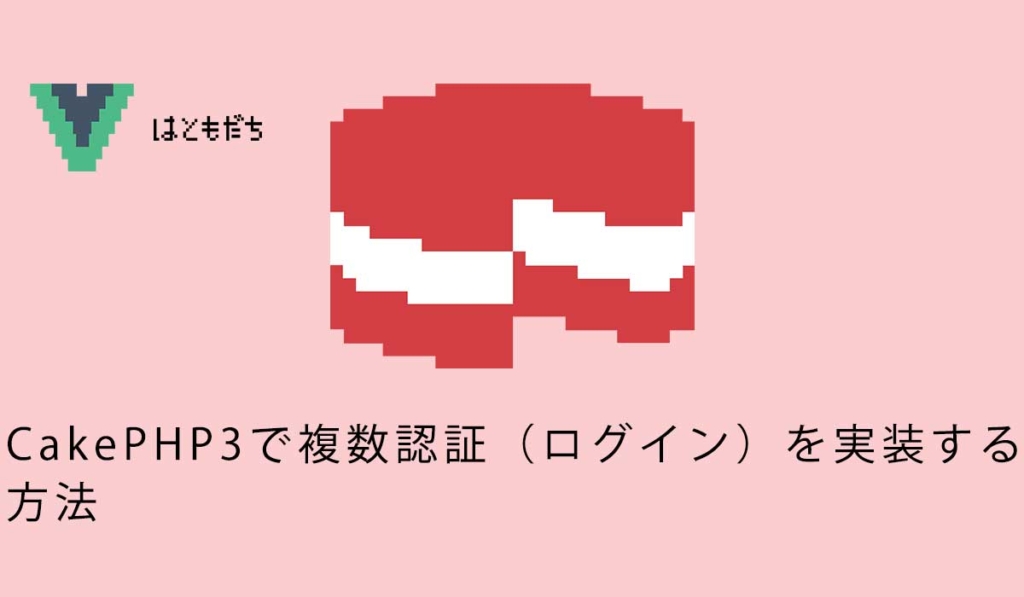
407 回閲覧されました
みなさんこんにちは、jonioです。
今回はCakePHP3で複数認証する方法のメモになります。
CakePHPのバージョン
3.6.15です。
複数認証するテーブル
usersテーブルとcompaniesテーブルの両方でログインができるようにします。
AppController.php
下記の記述をします。
<?php
/**
* CakePHP(tm) : Rapid Development Framework (https://cakephp.org)
* Copyright (c) Cake Software Foundation, Inc. (https://cakefoundation.org)
*
* Licensed under The MIT License
* For full copyright and license information, please see the LICENSE.txt
* Redistributions of files must retain the above copyright notice.
*
* @copyright Copyright (c) Cake Software Foundation, Inc. (https://cakefoundation.org)
* @link https://cakephp.org CakePHP(tm) Project
* @since 0.2.9
* @license https://opensource.org/licenses/mit-license.php MIT License
*/
namespace App\Controller;
use Cake\Controller\Controller;
use Cake\Event\Event;
/**
* Application Controller
*
* Add your application-wide methods in the class below, your controllers
* will inherit them.
*
* @link https://book.cakephp.org/3.0/en/controllers.html#the-app-controller
*/
class AppController extends Controller
{
/**
* Initialization hook method.
*
* Use this method to add common initialization code like loading components.
*
* e.g. `$this->loadComponent('Security');`
*
* @return void
*/
public function initialize()
{
parent::initialize();
$this->loadComponent('RequestHandler');
$this->loadComponent('Flash');
//ここから追加
$this->loadComponent('Auth', [
'authenticate' => [
'Form' => [
'fields' => [
'username' => 'email',
'password' => 'password'
],
'userModel' => 'Users'
]
],
'authError' => 'You are not authorized to access that location.',
'unauthorizedRedirect' => $this->referer(),
]);
//ここまで追加
/*
* Enable the following components for recommended CakePHP security settings.
* see https://book.cakephp.org/3.0/en/controllers/components/security.html
*/
//$this->loadComponent('Security');
//$this->loadComponent('Csrf');
}
//ここから追加
public function beforeFilter(Event $event)
{
parent::beforeFilter($event);
$controller = $this->request->getParam('controller');
if ($controller == 'Companies') {
$this->Auth->setConfig('authenticate', [
'Form' => [
'userModel' => 'Companies',
'fields' => [
'username' => 'email',
'password' => 'password'
]
]
]);
$this->Auth->setConfig('loginAction', ['controller' => 'Companies', 'action' => 'login']);
} elseif ($controller == 'Users') {
$this->Auth->setConfig('authenticate', [
'Form' => [
'userModel' => 'Users',
'fields' => [
'username' => 'email',
'password' => 'password'
]
]
]);
$this->Auth->setConfig('loginAction', ['controller' => 'Users', 'action' => 'login']);
}
}
//ここまで追加
}
49行目〜61行目はデフォルトのログインの設定でこれを書かないとログインが動作しません。
そしてログインをするコントローラーを分ける為に81行目〜105行目があります。
81行目〜93行目はcompaniesテーブルでログインする場合です。
93行目〜105行目はusersテーブルでログインする場合です。
モデル
パスワードのハッシュ化をします。
「CakePHPのプロジェクト > src > Model > Entity > 〜.php」に記述します。
今回はCompany.php のコードを掲載しますがUser.phpも同じ記述をします。
<?php
namespace App\Model\Entity;
use Cake\ORM\Entity;
use Cake\Auth\DefaultPasswordHasher; //この行を追加
/**
* Company Entity
*
* @property int $id
* @property string $name
* @property string $email
* @property string $password
* @property \Cake\I18n\FrozenTime $created
* @property \Cake\I18n\FrozenTime $modified
*/
class Company extends Entity
{
//ここから追加
protected function _setPassword($password)
{
return (new DefaultPasswordHasher)->hash($password);
}
//ここまで追加
コントローラー・ビュー
コントローラー・ビューのコードは同じです。
//コントローラー
public function login()
{
if ($this->request->is('post')) {
$user = $this->Auth->identify();
if ($user) {
$this->Auth->setUser($user);
return $this->redirect(['action' => 'index']);
}
$this->Flash->error(__('Invalid username or password, try again'));
}
}
public function logout()
{
return $this->redirect($this->Auth->logout());
}
//ビュー
<div class="form">
<?= $this->Flash->render() ?>
<h3>Login</h3>
<?= $this->Form->create() ?>
<fieldset>
<legend><?= __('Please enter your username and password') ?></legend>
<?= $this->Form->control('email', ['required' => true]) ?>
<?= $this->Form->control('password', ['required' => true]) ?>
</fieldset>
<?= $this->Form->submit(__('Login')); ?>
<?= $this->Form->end() ?>
</div>
これで完成です。